Does the Application Class Get Called Again on Version Upgrades
English | Português | 简体中文 | Español | 한국어
A wrapper effectually InheritedWidget to make them easier to utilise and more reusable.
Past using provider
instead of manually writing InheritedWidget, you get:
- simplified allocation/disposal of resource
- lazy-loading
- a vastly reduced boilerplate over making a new grade every fourth dimension
- devtool friendly – using Provider, the state of your awarding will be visible in the Palpitate devtool
- a common mode to consume these InheritedWidgets (Meet Provider.of/Consumer/Selector)
- increased scalability for classes with a listening mechanism that grows exponentially in complexity (such every bit ChangeNotifier, which is O(Due north) for dispatching notifications).
To read more than almost a provider
, see its documentation.
See also:
- The official Flutter state direction documentation, which showcases how to use
provider
+ ChangeNotifier - flutter architecture sample, which contains an implementation of that app using
provider
+ ChangeNotifier - flutter_bloc and Mobx, which uses a
provider
in their architecture
-
initialData
for bothFutureProvider
andStreamProvider
is at present required.To migrate, what used to exist:
FutureProvider<int>( create: (context) => Time to come.value(42), kid: MyApp(), ) Widget build(BuildContext context) { final value = context.watch<int>(); render Text('$value'); }
is at present:
FutureProvider<int?>( initialValue: null, create: (context) => Future.value(42), child: MyApp(), ) Widget build(BuildContext context) { // exist sure to specify the ? in spotter<int?> terminal value = context.spotter<int?>(); return Text('$value'); }
-
ValueListenableProvider
is removedTo drift, you can instead use
Provider
combined withValueListenableBuilder
:ValueListenableBuilder<int>( valueListenable: myValueListenable, builder: (context, value, _) { render Provider<int>.value( value: value, child: MyApp(), ); } )
Exposing a new object example
Providers allow you to not simply expose a value, only also create, mind, and dispose of information technology.
To expose a newly created object, use the default constructor of a provider. Do not utilise the .value
constructor if you want to create an object, or yous may otherwise accept undesired side effects.
Run into this StackOverflow answer which explains why using the .value
constructor to create values is undesired.
- DO create a new object inside
create
.
Provider( create: (_) => MyModel(), child: ... )
- DON'T use
Provider.value
to create your object.
ChangeNotifierProvider.value( value: MyModel(), child: ... )
-
DON'T create your object from variables that tin can modify over time.
In such a situation, your object would never update when the value changes.
int count; Provider( create: (_) => MyModel(count), child: ... )
If you want to pass variables that can alter over fourth dimension to your object, consider using ProxyProvider
:
int count; ProxyProvider0( update: (_, __) => MyModel(count), child: ... )
Note:
When using the create
/update
callback of a provider, it is worth noting that this callback is chosen lazily by default.
This means that until the value is requested at least in one case, the create
/update
callbacks won't exist called.
This behavior tin can be disabled if yous desire to pre-compute some logic, using the lazy
parameter:
MyProvider( create: (_) => Something(), lazy: false, )
Reusing an existing object case:
If y'all already take an object instance and want to expose information technology, it would be all-time to use the .value
constructor of a provider.
Failing to do then may telephone call your object dispose
method when it is still in use.
- DO utilise
ChangeNotifierProvider.value
to provide an existing ChangeNotifier.
MyChangeNotifier variable; ChangeNotifierProvider.value( value: variable, child: ... )
- DON'T reuse an existing ChangeNotifier using the default constructor
MyChangeNotifier variable; ChangeNotifierProvider( create: (_) => variable, kid: ... )
The easiest way to read a value is by using the extension methods on [BuildContext]:
-
context.watch<T>()
, which makes the widget listen to changes onT
-
context.read<T>()
, which returnsT
without listening to it -
context.select<T, R>(R cb(T value))
, which allows a widget to listen to merely a small part ofT
.
Ane tin also use the static method Provider.of<T>(context)
, which will behave similarly to watch
and when the listen
parameter is set to fake
like Provider.of<T>(context, listen: false)
, and then it volition behave similarly to read
.
It'southward worth noting that context.read<T>()
won't make a widget rebuild when the value changes and it cannot exist chosen within StatelessWidget.build
/State.build
. On the other hand, it can be freely called outside of these methods.
These methods volition look up in the widget tree starting from the widget associated with the BuildContext
passed and will return the nearest variable of type T
found (or throw if cypher is found).
This operation is O(1). It doesn't involve walking in the widget tree.
Combined with the commencement example of exposing a value, this the widget will read the exposed String
and render "Hello Earth."
class Home extends StatelessWidget { @override Widget build(BuildContext context) { return Text( // Don't forget to pass the type of the object you lot desire to obtain to `watch`! context.watch<String>(), ); } }
Alternatively, instead of using these methods, nosotros tin can use Consumer and Selector.
These can be useful for performance optimizations or when information technology is difficult to obtain a BuildContext
descendant of the provider.
See the FAQ or the documentation of Consumer and Selector for more data.
Sometimes, nosotros may want to support cases where a provider does non be. An example would be for reusable widgets that could be used in various locations, including exterior of a provider.
To do so, when calling context.lookout man
/context.read
, make the generic blazon nullable. Such that instead of:
context.watch<Model>()
which will throw a ProviderNotFoundException
if no matching providers are found, do:
context.watch<Model?>()
which volition attempt to obtain a matching provider. But if none are institute, null
volition be returned instead of throwing.
When injecting many values in big applications, Provider
can quickly become pretty nested:
Provider<Something>( create: (_) => Something(), child: Provider<SomethingElse>( create: (_) => SomethingElse(), child: Provider<AnotherThing>( create: (_) => AnotherThing(), child: someWidget, ), ), ),
To:
MultiProvider( providers: [ Provider<Something>(create: (_) => Something()), Provider<SomethingElse>(create: (_) => SomethingElse()), Provider<AnotherThing>(create: (_) => AnotherThing()), ], child: someWidget, )
The behavior of both examples is strictly the same. MultiProvider
only changes the appearance of the code.
Since the 3.0.0, in that location is a new kind of provider: ProxyProvider
.
ProxyProvider
is a provider that combines multiple values from other providers into a new object and sends the result to Provider
.
That new object will then exist updated whenever one of the provider we depend on gets updated.
The post-obit example uses ProxyProvider
to build translations based on a counter coming from another provider.
Widget build(BuildContext context) { render MultiProvider( providers: [ ChangeNotifierProvider(create: (_) => Counter()), ProxyProvider<Counter, Translations>( update: (_, counter, __) => Translations(counter.value), ), ], child: Foo(), ); } class Translations { const Translations(this._value); last int _value; Cord get title => 'You clicked $_value times'; }
Information technology comes under multiple variations, such equally:
-
ProxyProvider
vsProxyProvider2
vsProxyProvider3
, ...That digit after the class name is the number of other providers that
ProxyProvider
depends on. -
ProxyProvider
vsChangeNotifierProxyProvider
vsListenableProxyProvider
, ...They all work similarly, only instead of sending the result into a
Provider
, aChangeNotifierProxyProvider
will send its value to aChangeNotifierProvider
.
Can I audit the content of my objects?
Palpitate comes with a devtool that shows what the widget tree is at a given moment.
Since providers are widgets, they are also visible in that devtool:
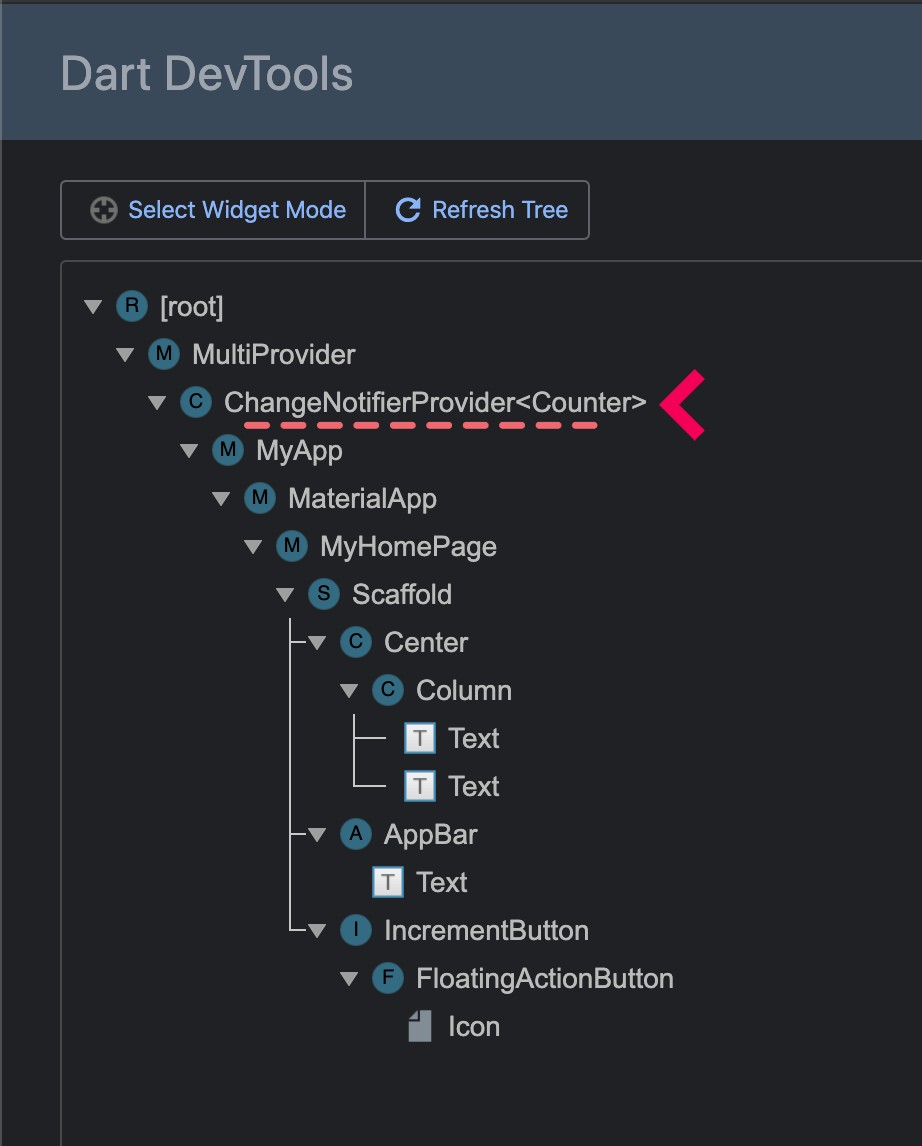
From at that place, if yous click on one provider, you lot will be able to come across the value information technology exposes:

(screenshot of the devtools using the case
folder)
The devtool just shows "Instance of MyClass". What tin can I practice?
Past default, the devtool relies on toString
, which defaults to "Instance of MyClass".
To accept something more useful, yous have 2 solutions:
-
apply the Diagnosticable API from Flutter.
For nearly cases, I will employ DiagnosticableTreeMixin on your objects, followed by a custom implementation of debugFillProperties.
grade MyClass with DiagnosticableTreeMixin { MyClass({this.a, this.b}); final int a; final String b; @override void debugFillProperties(DiagnosticPropertiesBuilder properties) { super.debugFillProperties(properties); // list all the properties of your class here. // See the documentation of debugFillProperties for more data. properties.add together(IntProperty('a', a)); properties.add(StringProperty('b', b)); } }
-
Override
toString
.If you cannot apply DiagnosticableTreeMixin (like if your class is in a package that does non depend on Palpitate), then y'all can override
toString
.This is easier than using DiagnosticableTreeMixin simply is less powerful: You lot will not be able to expand/collapse the details of your object.
class MyClass with DiagnosticableTreeMixin { MyClass({this.a, this.b}); final int a; terminal String b; @override String toString() { render '$runtimeType(a: $a, b: $b)'; } }
I take an exception when obtaining Providers inside initState
. What tin can I do?
This exception happens because you lot're trying to listen to a provider from a life-bike that will never ever be called again.
Information technology ways that you either should use another life-cycle (build
), or explicitly specify that you do not intendance most updates.
As such, instead of:
initState() { super.initState(); print(context.spotter<Foo>().value); }
y'all can practice:
Value value; Widget build(BuildContext context) { final value = context.lookout man<Foo>.value; if (value != this.value) { this.value = value; print(value); } }
which will print value
whenever information technology changes (and only when it changes).
Alternatively, you can practise:
initState() { super.initState(); print(context.read<Foo>().value); }
Which will print value
once and ignore updates.
How to handle hot-reload on my objects?
You can make your provided object implement ReassembleHandler
:
class Example extends ChangeNotifier implements ReassembleHandler { @override void reassemble() { impress('Did hot-reload'); } }
And then used typically with provider
:
ChangeNotifierProvider(create: (_) => Example()),
I use ChangeNotifier, and I have an exception when I update it. What happens?
This likely happens because you are modifying the ChangeNotifier from one of its descendants while the widget tree is building.
A typical situation where this happens is when starting an http asking, where the hereafter is stored within the notifier:
initState() { super.initState(); context.read<MyNotifier>().fetchSomething(); }
This is not allowed because the country update is synchronous.
This ways that some widgets may build before the mutation happens (getting an quondam value), while other widgets will build after the mutation is complete (getting a new value). This could crusade inconsistencies in your UI and is therefore non allowed.
Instead, y'all should perform that mutation in a place that would affect the entire tree equally:
-
straight inside the
create
of your provider/constructor of your model:class MyNotifier with ChangeNotifier { MyNotifier() { _fetchSomething(); } Time to come<void> _fetchSomething() async {} }
This is useful when there's no "external parameter".
-
asynchronously at the terminate of the frame:
initState() { super.initState(); Future.microtask(() => context.read<MyNotifier>().fetchSomething(someValue); ); }
It is slightly less ideal, just allows passing parameters to the mutation.
Do I have to employ ChangeNotifier for complex states?
No.
Yous tin can use any object to represent your state. For example, an alternating architecture is to use Provider.value()
combined with a StatefulWidget
.
Here'due south a counter example using such architecture:
class Example extends StatefulWidget { const Instance({Key fundamental, this.kid}) : super(cardinal: cardinal); final Widget child; @override ExampleState createState() => ExampleState(); } class ExampleState extends Land<Example> { int _count; void increase() { setState(() { _count++; }); } @override Widget build(BuildContext context) { return Provider.value( value: _count, child: Provider.value( value: this, child: widget.child, ), ); } }
where we can read the state by doing:
return Text(context.watch<int>().toString());
and modify the state with:
return FloatingActionButton( onPressed: () => context.read<ExampleState>().increment(), child: Icon(Icons.plus_one), );
Alternatively, you lot tin can create your ain provider.
Tin can I make my Provider?
Yep. provider
exposes all the pocket-size components that make a fully-fledged provider.
This includes:
-
SingleChildStatelessWidget
, to make any widget works withMultiProvider
.
This interface is exposed as function ofpackage:provider/single_child_widget
-
InheritedProvider, the generic
InheritedWidget
obtained when doingcontext.watch
.
Here's an case of a custom provider to use ValueNotifier
every bit the state: https://gist.github.com/rrousselGit/4910f3125e41600df3c2577e26967c91
My widget rebuilds too often. What can I do?
Instead of context.watch
, y'all tin can use context.select
to listen only to the specific set of properties on the obtained object.
For example, while yous tin write:
Widget build(BuildContext context) { last person = context.lookout man<Person>(); return Text(person.name); }
It may cause the widget to rebuild if something other than name
changes.
Instead, y'all tin use context.select
to listen but to the name
property:
Widget build(BuildContext context) { final name = context.select((Person p) => p.name); return Text(proper noun); }
This way, the widget won't unnecessarily rebuild if something other than name
changes.
Similarly, y'all can employ Consumer/Selector. Their optional child
argument allows rebuilding only a particular part of the widget tree:
Foo( child: Consumer<A>( architect: (_, a, child) { return Bar(a: a, kid: child); }, child: Baz(), ), )
In this instance, merely Bar
will rebuild when A
updates. Foo
and Baz
won't unnecessarily rebuild.
Tin can I obtain two different providers using the same type?
No. While you tin can have multiple providers sharing the aforementioned blazon, a widget will be able to obtain but one of them: the closest ancestor.
Instead, information technology would help if you explicitly gave both providers a unlike blazon.
Instead of:
Provider<String>( create: (_) => 'England', child: Provider<String>( create: (_) => 'London', kid: ..., ), ),
Adopt:
Provider<Country>( create: (_) => Land('England'), child: Provider<Metropolis>( create: (_) => City('London'), kid: ..., ), ),
Can I consume an interface and provide an implementation?
Yes, a type hint must be given to the compiler to signal the interface will be consumed, with the implementation provided in create.
abstract class ProviderInterface with ChangeNotifier { ... } grade ProviderImplementation with ChangeNotifier implements ProviderInterface { ... } grade Foo extends StatelessWidget { @override build(context) { final provider = Provider.of<ProviderInterface>(context); render ... } } ChangeNotifierProvider<ProviderInterface>( create: (_) => ProviderImplementation(), child: Foo(), ),
provider
exposes a few different kinds of "provider" for different types of objects.
The complete list of all the objects bachelor is hither
proper name | description |
---|---|
Provider | The most basic form of provider. It takes a value and exposes it, whatsoever the value is. |
ListenableProvider | A specific provider for Listenable object. ListenableProvider will heed to the object and ask widgets which depend on information technology to rebuild whenever the listener is called. |
ChangeNotifierProvider | A specification of ListenableProvider for ChangeNotifier. It volition automatically phone call ChangeNotifier.dispose when needed. |
ValueListenableProvider | Listen to a ValueListenable and only expose ValueListenable.value . |
StreamProvider | Listen to a Stream and betrayal the latest value emitted. |
FutureProvider | Takes a Future and updates dependents when the future completes. |
If you have a very big number of providers (150+), it is possible that some devices volition throw a StackOverflowError
because you stop-upward building too many widgets at once.
In this situation, you have a few solutions:
-
If your application has a splash-screen, try mounting your providers over fourth dimension instead of all at once.
You could do:
MultiProvider( providers: [ if (step1) ...[ <lots of providers>, ], if (step2) ...[ <some more providers> ] ], )
where during your splash screen animation, you lot would do:
bool step1 = false; bool step2 = false; @override initState() { super.initState(); Future(() { setState(() => step1 = true); Time to come(() { setState(() => step2 = true); }); }); }
-
Consider opting out of using
MultiProvider
.MultiProvider
works past adding a widget between every providers. Non usingMultiProvider
can increase the limit before aStackOverflowError
is reached.
Source: https://pub.dev/packages/provider
0 Response to "Does the Application Class Get Called Again on Version Upgrades"
Postar um comentário